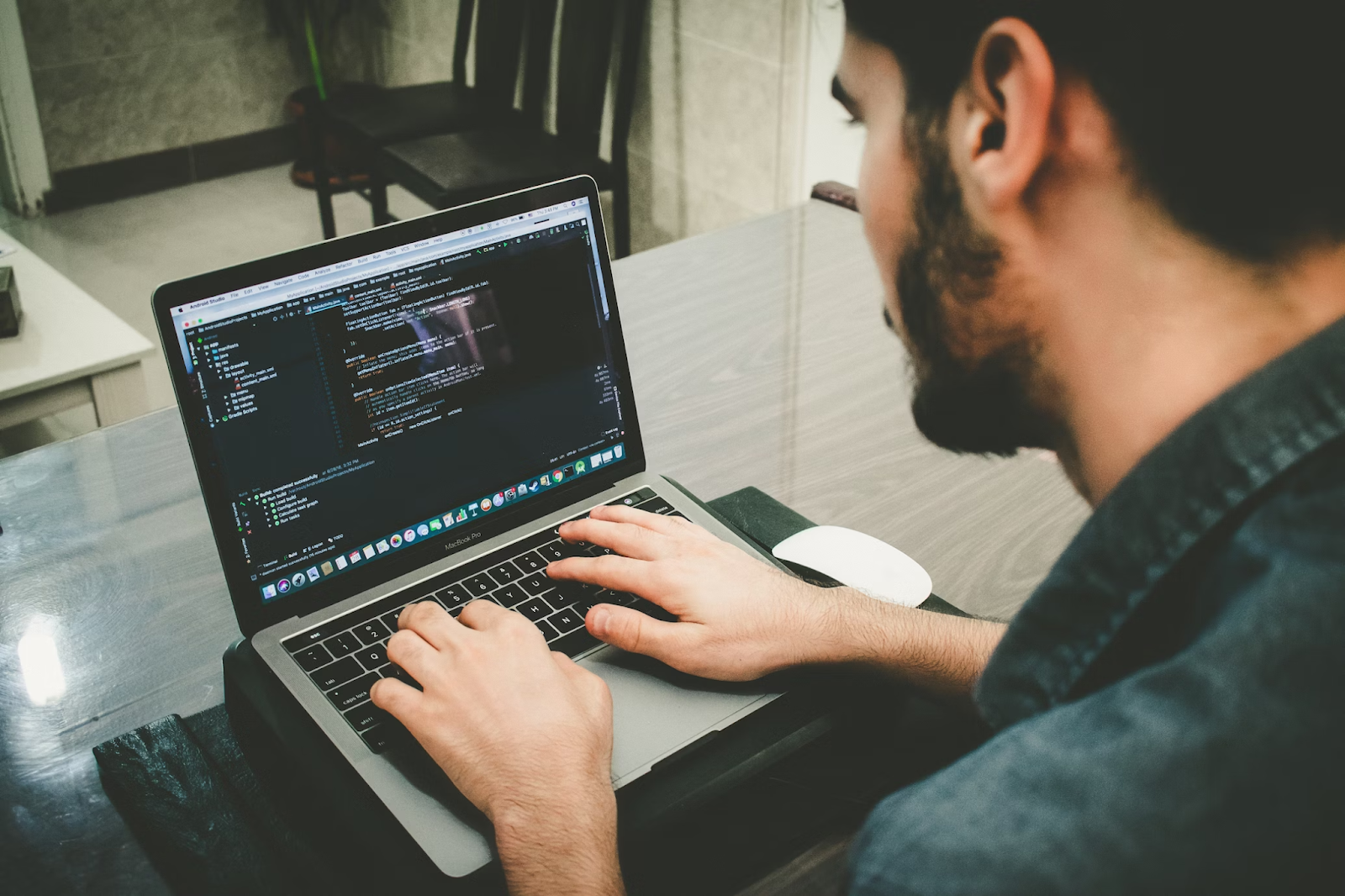
Table of Contents
Introduction
In the fast-paced world of container orchestration, Kubernetes has emerged as a dominant force, streamlining the deployment and management of containerized applications. As Kubernetes environments continue to advance, the demand for robust tools that enhance observability, security, and performance becomes increasingly crucial. Enter eBPF (Extended Berkeley Packet Filter), a dynamic and versatile technology that is transforming the Kubernetes landscape.
Understanding the Basics of eBPF
At its core, eBPF serves as a programmable interface within the Linux kernel, offering a pathway for efficient and low-overhead instrumentation. Unlike traditional tracing methods, eBPF enables developers to inject custom code into the kernel dynamically without necessitating modifications or recompilation. This unique capability opens the door to a myriad of possibilities, making it an ideal fit for augmenting Kubernetes environments.
eBPF in Action: Observability
One of the key areas that shines in eBPF Kubernetes is observability. By leveraging eBPF, developers can gain unprecedented insights into the performance of their applications and infrastructure. Through the dynamic attachment of probes to various kernel events, such as function calls or network activities, eBPF facilitates real-time monitoring without incurring the overhead associated with traditional monitoring solutions.
Let’s delve into a straightforward eBPF example for observability. The following code traces the execution of a specific function in the kernel:
#include <uapi/linux/ptrace.h>
BPF_TRACE_PROBE(kernel_function) {
bpf_trace_printk("Function called!\\n");
return 0;
}
This concise eBPF program prints a message each time the specified kernel function is called. When implemented, it provides real-time insights into kernel-level activities, aiding in troubleshooting and optimizing resource utilization.
Security Enhancements with eBPF
Security is a paramount concern in any Kubernetes deployment, and eBPF offers a robust set of tools to fortify containerized environments. By applying eBPF-powered security policies, administrators can create fine-grained controls over network traffic, thereby reducing the attack surface. The capability to filter and analyze network packets in real-time allows for the rapid detection and mitigation of security incidents.
Consider the following eBPF code snippet for network traffic analysis:
#include <uapi/linux/bpf.h>
#include <uapi/linux/if_ether.h>
#include <uapi/linux/ip.h>
BPF_TABLE("hash", u64, u64, packet_counts, 256);
BPF_TRACE_PROBE(net_receive) {
struct ethhdr *eth = bpf_hdr_pointer(ctx, 0);
u64 *count;
// Count packets based on source MAC address
count = packet_counts.lookup_or_try_init(ð->h_source, &ZERO);
if (count) {
(*count)++;
}
return 0;
}
In this example, eBPF tracks the number of received packets based on the source MAC address, fortifying Kubernetes security by providing real-time insights into network activities.
Performance Optimization Through eBPF
Efficient resource utilization is critical for achieving optimal performance in Kubernetes environments. eBPF emerges as a key player in this pursuit, offering a lightweight and non-intrusive means of collecting performance metrics. By tapping into kernel-level events, such as CPU usage, memory allocations, and disk I/O, eBPF empowers administrators to pinpoint performance bottlenecks and fine-tune their deployments.
Let’s explore a concise eBPF code snippet for monitoring CPU usage:
#include <uapi/linux/bpf.h>
#include <uapi/linux/perf_event.h>
BPF_PERF_OUTPUT(cpu_usage);
BPF_TRACE_PROBE(raw_syscalls_sys_enter) {
u64 pid_tgid = bpf_get_current_pid_tgid();
u32 tgid = pid_tgid >> 32;
// Attach to the task and measure CPU usage
struct task_struct *task = (struct task_struct *)bpf_get_current_task();
u64 timestamp = bpf_ktime_get_ns();
cpu_usage.perf_submit(ctx, ×tamp, sizeof(timestamp));
return 0;
}
This eBPF program measures CPU usage by timestamping each system call entry. Integrating such metrics into Kubernetes environments aids in identifying and mitigating performance issues, ensuring smooth application deployments.
Real-Time Networking Insights with eBPF
Networking is a cornerstone of Kubernetes, and eBPF provides invaluable tools for gaining real-time insights into network activities. With eBPF, administrators can create custom monitoring solutions to track network packets, detect anomalies, and optimize communication between microservices.
Consider the following eBPF code snippet for tracking TCP connection establishment:
#include <uapi/linux/bpf.h>
#include <uapi/linux/if_ether.h>
#include <uapi/linux/ip.h>
#include <uapi/linux/tcp.h>
BPF_HASH(connections, struct sock *, u64);
BPF_TRACE_PROBE(tcp_v4_connect) {
struct sock *sk = (struct sock *)ctx->skp;
u64 timestamp = bpf_ktime_get_ns();
// Track TCP connection establishment
connections.update(&sk, ×tamp);
return 0;
}
In this example, eBPF captures the timestamp of each TCP connection establishment event, offering real-time insights into networking activities within the Kubernetes cluster.
Automating Kubernetes Operations with eBPF
In addition to its capabilities in observability, security and performance optimization, eBPF serves as a powerful tool for automating routine operations in Kubernetes environments. Automation is a key pillar for managing the complexity of container orchestration at scale, and eBPF facilitates this by providing real-time insights that can be leveraged for intelligent automation.
Consider a scenario where eBPF is employed to automate the scaling of Kubernetes pods based on network traffic patterns:
#include <uapi/linux/bpf.h>
#include <uapi/linux/if_ether.h>
#include <uapi/linux/ip.h>
BPF_HASH(pod_scaling, u32, u64);
BPF_TRACE_PROBE(net_receive) {
struct ethhdr *eth = bpf_hdr_pointer(ctx, 0);
u32 pod_id = /* Calculate or extract pod identifier based on traffic */;
u64 *packet_count;
// Update packet count for the respective pod
packet_count = pod_scaling.lookup_or_try_init(&pod_id, &ZERO);
if (packet_count) {
(*packet_count)++;
// Automatically scale the pod if a threshold is reached
if (*packet_count > THRESHOLD) {
// Implement logic for scaling the pod
// ...
}
}
return 0;
}
In this example, eBPF dynamically monitors network traffic and increments the packet count for each associated pod. When a predefined threshold (THRESHOLD) is reached, the eBPF program triggers an automated scaling action. This level of automation, driven by real-time insights provided by eBPF, enhances the efficiency of Kubernetes operations by dynamically responding to changes in workload demands.
The automation capabilities of eBPF extend beyond scaling. eBPF programs can be tailored to automate tasks such as resource allocation, load balancing, and even self-healing mechanisms. By leveraging eBPF’s ability to interact with the Kubernetes API and other components, administrators can craft intelligent automation solutions that adapt to the dynamic nature of containerized workloads.
#include <uapi/linux/bpf.h>
BPF_TABLE("hash", u32, u64, resource_allocation);
BPF_TRACE_PROBE(workload_change_event) {
u32 pod_id = /* Extract pod identifier from the event */;
// Dynamically allocate resources based on workload changes
resource_allocation.update(&pod_id, &NEW_RESOURCE_VALUES);
// Implement logic for applying resource changes to the Kubernetes API
// ...
return 0;
}
In this hypothetical example, eBPF reacts to workload changes and updates resource allocation parameters dynamically. This information can then be communicated to the Kubernetes API for automated adjustment of resources assigned to the respective pods.
Challenges and Considerations
While eBPF brings a plethora of advantages to Kubernetes environments, it is essential to be mindful of potential challenges. Integration with existing tooling, ensuring compatibility across kernel versions, and understanding the intricacies of eBPF programming are aspects that require careful consideration.
Additionally, as eBPF continues to evolve, staying abreast of updates and community-driven developments becomes imperative. The vibrant open-source ecosystem around eBPF ensures a wealth of resources, but it also demands a proactive approach to keep pace with advancements and best practices.
Conclusion
In the ever-evolving realm of Kubernetes, harnessing the power of eBPF proves to be a strategic move for organizations seeking enhanced observability, fortified security, and optimized performance. By seamlessly integrating with the kernel, eBPF offers a versatile toolkit that empowers developers and administrators to unlock new levels of efficiency and control.
As Kubernetes environments become more complex, the need for agile and robust solutions becomes paramount. eBPF, with its programmability and low overhead, stands as a beacon of innovation, illuminating the path to a more resilient, secure, and performant Kubernetes ecosystem. As organizations continue to unravel the capabilities of eBPF, the journey towards unlocking its full potential is just beginning.